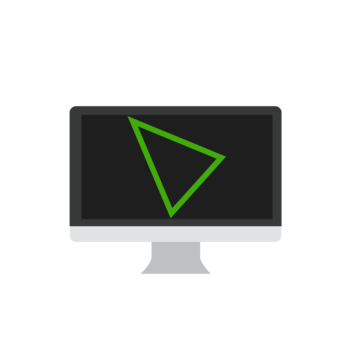
Using the Pythagorean theorem (programming)

Upgrade for more content
True or false? A triangle is right-angled if there is a 90-degree angle.
This is a triangle. How do we know if it is a right-angled triangle? We can check if it has a 90 degree angle by using Pythagoras' Theorem. It is only in a right-angled triangle that this relationship applies c² = a² + b² To test this, we insert the lengths of the sides into the equation, and see whether it's true - whether the left side is equal to the right side. Now we are going to make a program that tests if any triangle is right-angled or not.
To complete the calculation, we need the lengths of the three sides of the triangle. So the first three instructions in the program will be: Note side 1 Note side 2 Note side 3 What should the program do next? In order to apply Pythagoras' Theorem, we need to check which side is the longest. We call it c. The other two sides become a and b IF: c² = a² + b² THEN: this means it is a right-angled triangle ELSE: means that it is not.
But this bit? Find out which side is the longest. How shall we do that? We can use three if-statements, like this! In these rows we check if side 1 is the longest, then we create variable c, and give it the value of the length of side 1.
In these rows we do the same process with side 2. And in these rows we test side 3. Here the program got 'no' as an answer to all three if-statements, so no side is longer than the other two, and the triangle is not right-angled. So we output that it is not a right-angled triangle, and the program ends. If we know that variable c is the longest side of the triangle, the two shorter sides must be: variable a, and variable b.
Which of the short sides becomes variable a or variable b does not matter. Because a² + b² is in fact the same as b² + a² Is our pseudocode ready now? Not really. In order to make it clear which parts of the code are associated with other parts, you usually link the rows that belong together. Rows 5 to 7 are there because they are connected to the if-statement in row 4.
These rows are executed only when the if-statement in row 4 is true. The same with rows 9 to 11 and 13 to 15 and row 20. Rows 17, 18, and 22 are connected to the else-statement. Look here: this is the part of the code that solves the task itself. That is the algorithm.
IF c² = a² + b² A flowchart may be helpful to get an overview of the program. We draw one box for each step. Create and give values to the variables s1 , s2 and s3. If s1 is the greatest, create variable c and assign the value from s1. Variable a gets the value from s2 and variable b gets the value from s3.
Do the same with s2. And with s3. IF no side is longer than the other two, THEN it is not a right-angled triangle: end program. Now we know which side is the longest and it is called c. Variable a2 gets the value a times a Variable b2 gets the value b times b Variable c2 gets the value c times c Finally, we can test whether: c2 = a2 + b2 IF that is true, THEN the program outputs 'yes', ELSE the program outputs 'no'.
Now, it's your turn again, to do some programming!